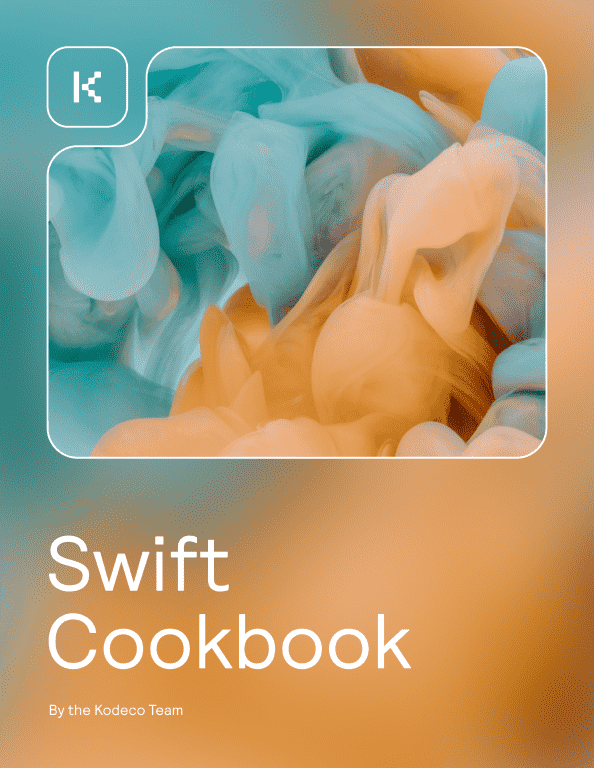
Swift Cookbook
The Swift Cookbook is a collection of common Swift language idioms and best practices that you can quickly refer to while coding. By Team Kodeco.
Who is this for?
The Swift Cookbook is for anyone writing Swift code who needs a quick refresh of a particular syntax or technique. As a reference, each segment is independent and you are encouraged to skip around as you need.
Covered concepts
The Swift Cookbook covers common Swift language idioms and best practices. It is mainly concerned with fundamental language feature and the standard library. It also may evolve to cover generally available Swift packages over time.
The Swift Cookbook is a collection of common Swift language idioms and best practices that you can quickly refer to while coding.
moreSwift Variables & Constants
This section covers the fundamental concepts of variables and constants in the language, including how to declare and initialize them, as well as their mutability and type annotations.
One of the key takeaways from this section is the importance of understanding the differences between value types and reference types. This knowledge will help you make informed decisions about when to use variables and when to use constants, and can have a significant impact on the performance and behavior of your code.
Additionally, the section covers advanced topics such as lazy initialization and the ternary operator, providing you with powerful tools to write more efficient and readable code. By the end of this section, you’ll have a solid understanding of how to use variables and constants in Swift, and be well on your way to becoming a proficient Swift developer.
Swift Data Types
Swift Data Types is an essential section of the Swift Cookbook that covers the different types of data that can be used in a Swift program. Understanding these data types is crucial for any programmer, as it forms the building blocks of any application.
The section covers various data types such as integers, floating-point numbers, boolean values, strings, tuples, arrays, dictionaries, sets, enumerations, and optionals. Understanding how to use these data types effectively can help you create more efficient and expressive code, making it easier to read, understand and maintain.
In this section, you’ll learn how to use each data type in a way that is efficient, clear and easy to understand. You’ll also learn how to use these data types together to create complex data structures and how to work with these data types in different contexts. This section is a must-read for anyone looking to master the Swift programming language.
Swift Optionals
The “Swift Optionals” section of the Swift Cookbook is an essential guide for anyone looking to master the use of optionals in the Swift programming language. Understanding optionals is crucial for writing safe and robust code in Swift and this section provides a comprehensive introduction to the topic.
This section covers the basics of optionals, including how to define and work with them, as well as advanced techniques such as optional binding, optional chaining and the nil-coalescing operator. You’ll also learn about more advanced features of optionals, such as the optional ternary operator, implicitly unwrapped optionals and optional map and flatMap. Additionally, you’ll learn how to use optional comparison operators and guard statement with optionals.
Optionals are a powerful feature in Swift and this section will help you understand how to use them effectively and safely. By the end of this section, you’ll have a solid understanding of optionals and how to use them to write safer and more expressive code. This section is a must-read for anyone looking to become proficient in the Swift programming language.
Swift Control Flow
The “Swift Control Flow” section of the Swift Cookbook is an essential guide for anyone looking to master the use of control flow statements in the Swift programming language. Understanding control flow is crucial for writing efficient and expressive code in Swift and this section provides a comprehensive introduction to the topic.
This section covers the basics of control flow statements, including if-else statements, switch statements, while loops, repeat-while loops, for loops, and for-in loops. You’ll also learn about more advanced features of control flow, such as the use of break and continue in loops, guard statements, fallthrough in switch condition and labeled statements.
By mastering control flow statements, you can write more expressive and efficient code that’s easy to read, understand and maintain. This section will help you understand how to use control flow statements effectively and safely. By the end of this section, you’ll have a solid understanding of control flow statements and how to use them to write safer and more expressive code. This section is a must-read for anyone looking to become proficient in the Swift programming language.
Swift Operators
The “Swift Operators” section of the Swift Cookbook is an essential guide for anyone looking to master the use of operators in the Swift programming language. Understanding operators is crucial for writing efficient and expressive code in Swift, and this section provides a comprehensive introduction to the topic.
This section covers the basics of operators, including arithmetic, comparison, logical and ternary conditional operator. You will also learn about more advanced features of operators, such as range operators, nil-coalescing operator, tuple comparison operator and custom operators. Additionally, you will learn how to use overloaded operators and bitwise operators in Swift.
Operators are a powerful feature in Swift, and this section will help you understand how to use them effectively and safely. By mastering operators, you can write more expressive and efficient code that is easy to read, understand and maintain. This section is a must-read for anyone looking to become proficient in the Swift programming language.
Swift Functions
The “Swift Functions” section of the Swift Cookbook is an essential guide for anyone looking to master the use of functions in the Swift programming language. Understanding functions is crucial for writing efficient, reusable and maintainable code, and this section provides a comprehensive introduction to the topic.
This section covers the basics of functions, including how to declare, call and pass parameters to them, and how to use return types. You’ll also learn about more advanced features of functions, such as external parameter names, default parameter values, variadic parameters, inout parameters, function types, higher-order functions and recursive functions.
Functions are a fundamental building block of any Swift program, and this section will help you understand how to use them effectively and safely. By mastering functions, you can write more expressive, efficient and maintainable code that is easy to read, understand and maintain. This section is a must-read for anyone looking to become proficient in the Swift programming language.
Swift Strings
The “Swift Strings” section of the Swift Cookbook is an essential guide for anyone looking to master the use of strings in the Swift programming language. Understanding strings is crucial for writing efficient, expressive, and maintainable code, and this section provides a comprehensive introduction to the topic.
This section covers the basics of strings, including how to create, modify and compare them, and how to use string interpolation. You’ll also learn about more advanced features of strings, such as string characters, indices, slicing, concatenation, formatting, encoding and regular expressions.
Strings are a fundamental data type in any programming language and this section will help you understand how to use them effectively and safely. By mastering strings, you can write more expressive, efficient and maintainable code that is easy to read, understand and maintain. This section is a must-read for anyone looking to become proficient in the Swift programming language.
Swift Tuples
The “Swift Tuples” section of the Swift Cookbook is an essential guide for anyone looking to master the use of tuples in the Swift programming language. Understanding tuples is crucial for writing efficient, expressive, and maintainable code, and this section provides a comprehensive introduction to the topic.
This section covers the basics of tuples, including how to create, use and access them, and how to decompose them. You’ll also learn about more advanced features of tuples, such as tuple comparison operators, named tuples, tuple as function return types, tuples in switch statements, tuple patterns, tuple shuffling and tuple splat.
Tuples are a powerful feature in Swift and this section will help you understand how to use them effectively and safely. By mastering tuples, you can write more expressive, efficient and maintainable code that is easy to read, understand and maintain. This section is a must-read for anyone looking to become proficient in the Swift programming language.
Swift Collections
The “Swift Collections” section of the Swift Cookbook is an essential guide for anyone looking to master the use of collections in the Swift programming language. Understanding collections is crucial for writing efficient, expressive and maintainable code, and this section provides a comprehensive introduction to the topic.
This section covers the basics of collections, including how to use arrays, dictionaries and sets, and how to access and manipulate their elements. You’ll also learn about more advanced features of collections, such as array and dictionary subscripts, array and dictionary methods, set methods, Collection protocol and Lazy collections. Additionally, you’ll learn how to use collection algorithms in Swift.
Collections are a fundamental building block of any Swift program, and this section will help you understand how to use them effectively and safely. By mastering collections, you can write more expressive, efficient and maintainable code that is easy to read, understand and maintain. This section is a must-read for anyone looking to become proficient in the Swift programming language.
Swift Enumerations
The “Swift Enumerations” section of the Swift Cookbook is an essential guide for anyone looking to master the use of enumerations in the Swift programming language. Understanding enumerations is crucial for writing efficient, expressive, and maintainable code, and this section provides a comprehensive introduction to the topic.
This section covers the basics of enumerations, including how to declare and use them, as well as more advanced features such as raw values, associated values, and recursive enumerations. You’ll also learn how to use enumeration methods and how to use enumerations in switch statements, iteration, collections, and optionals.
Enumerations are a powerful feature in Swift, and this section will help you understand how to use them effectively and safely. By mastering enumerations, you can write more expressive, efficient and maintainable code that is easy to read, understand and maintain. This section is a must-read for anyone looking to become proficient in the Swift programming language.
Swift Classes & Structures
The “Swift Classes & Structures” section of the Swift Cookbook is an essential guide for anyone looking to master the use of classes and structures in the Swift programming language. Understanding classes and structures is crucial for writing efficient, expressive, and maintainable code, and this section provides a comprehensive introduction to the topic.
This section covers the basics of classes and structures, including how to define, initialize, and use properties, methods and subscripts. You will also learn about more advanced features such as inheritance, polymorphism, extensions, and the difference between classes and structures.
Classes and structures are fundamental building blocks of any Swift program and this section will help you understand how to use them effectively and safely. By mastering classes and structures, you can write more expressive, efficient and maintainable code that is easy to read, understand and maintain. This section is a must-read for anyone looking to become proficient in the Swift programming language.
Static Functions & Properties
The “Static Functions & Properties” section of the Swift Cookbook is an essential guide for anyone looking to master the use of classes and structures in the Swift programming language. It covers the concepts of static functions and properties, their differences from instance functions and properties and how to use them to create singleton objects.
Static functions and properties are associated with the type, rather than instances of the type. This means that they can be called and accessed without having to create an instance of the type.
The section will give you a solid understanding of static functions and properties and how they can be used in different situations, including the creation of singleton objects, which are objects that have only one instance that is shared across the entire application.
By the end of this section, you’ll have a clear understanding of static functions and properties and how they can be used to write more efficient, maintainable, and scalable Swift code.
Swift Generics
The “Swift Generics” section of the Swift Cookbook is an essential guide for anyone looking to master the use of generics in the Swift programming language. Understanding generics is crucial for writing efficient, expressive and maintainable code, and this section provides a comprehensive introduction to the topic.
This section covers the basics of generics, including how to define generic types and constrain them with type constraints and associated types. You’ll also learn about more advanced features of generics such as where clauses, generic subscripts and how to use generics in extensions, protocols, structs, classes and recursive constraints.
Generics are a powerful feature in Swift and this section will help you understand how to use them effectively and safely. By mastering generics, you can write more expressive, efficient and maintainable code that is easy to read, understand and maintain. This section is a must-read for anyone looking to become proficient in the Swift programming language and looking for more advanced concepts in Swift.
Swift Protocols & Delegation
The “Swift Protocols & Delegation” section of the Swift Cookbook is an essential guide for anyone looking to master the use of protocols and delegation in the Swift programming language. Understanding protocols and delegation is crucial for writing efficient, expressive, and maintainable code, and this section provides a comprehensive introduction to the topic.
This section covers the basics of protocols, including how to define, adopt and conform to protocols, as well as more advanced features such as protocol inheritance, extension, protocol-oriented programming, protocol composition, associated types and protocol as types. You’ll also learn about self-requirement in protocols and the delegation pattern in Swift.
Protocols and delegation are powerful features in Swift and this section will help you understand how to use them effectively and safely. By mastering protocols and delegation, you can write more expressive, efficient and maintainable code that is easy to read, understand and maintain. This section is a must-read for anyone looking to become proficient in the Swift programming language.
Swift Memory Management
The “Swift Memory Management” section of the Swift Cookbook is an essential guide for anyone looking to understand and master memory management in the Swift programming language. Understanding memory management is crucial for writing efficient and reliable code, and this section provides a comprehensive introduction to the topic.
This section covers a variety of topics related to memory management in Swift, including Automatic Reference Counting (ARC), strong and weak references, unowned references, lazy initialization, memory leaks and debugging, ARC and closures, ARC and multithreading, retain cycles and weak references, Implicitly Unwrapped Optionals and Manual Memory Management using deinit.
You’ll learn how to use these features to write code that is efficient, reliable and easy to read, understand and maintain. This section is a must-read for anyone looking to become proficient in the Swift programming language and looking for more advanced concepts in Swift. Understanding memory management is crucial for writing code that is both efficient and safe, and this section will help you to achieve that goal.
Swift Error Handling
In the “Swift Error Handling” section of the book, readers will learn various techniques to handle errors in their code. The section covers topics such as using the do-catch statement, throwing and propagating errors, converting errors to optional values, and defining custom error types.
Readers will also learn about the Result type, which is a convenient way to handle errors, and how to use it in their own code. Additionally, the section covers important debugging techniques like asserting and preconditioning, and how to use the defer statement for cleanup.
Finally, the section also covers advanced error handling techniques like using try?, try! and retryable errors. By the end of this section, readers will have a solid understanding of error handling in Swift and be able to write robust and reliable code.
Swift Access Control
The “Swift Access Control” section of the book is all about understanding and managing the different levels of access that can be applied to elements in a Swift codebase. This includes understanding the basics of the four main access levels in Swift: private, file-private, internal and public. With a solid grasp of these access levels, you’ll be able to control which parts of your code are visible and accessible to other parts of the codebase.
Additionally, this section covers advanced topics such as creating custom access levels and controlling access to specific elements like initializers, subscripts, properties and methods. By the end of this section, you’ll be equipped with the knowledge and tools to create a codebase with a strong separation of concerns, making it easier to maintain and evolve over time.
Swift Closures
The “Swift Closures” section of the book provides a comprehensive guide on how to effectively use closures in Swift programming. Closures are self-contained blocks of code that can be passed around and executed at a later time, making them a powerful tool for writing clean and modular code.
The section starts by explaining the basics of defining and using closures in Swift, including the different syntax options available. It then covers advanced techniques such as passing closures as arguments, returning closures from functions and capturing values with closures.
The section also covers trailing closure syntax, which allows for a more concise way of passing closures as arguments. It also covers the use of escaping closures and autoclosures, which are used to handle asynchronous code.
The section also covers Higher-Order functions with closures, which are functions that take other functions as arguments or return them as results. The section also covers the use of map, filter and reduce with closures, which are functional programming techniques that allow you to perform complex operations on collections in a more concise and readable way.
This section is essential for any Swift developer looking to improve their skills and write more efficient and elegant code. Closures are a powerful feature of Swift and understanding how to use them effectively can greatly improve your ability to write clean, reusable and maintainable code.
Swift Operator Overloading
The “Swift Operator Overloading” section of the book covers how to customize the behavior of operators in Swift for your own custom types. By overloading operators, you can make your code more expressive and intuitive to read.
This section will teach you how to overload operators such as +, *, [], !, ??, && and || to work with your custom types. You’ll learn how to implement the Comparable protocol for custom types, how to overload the + operator for custom types, how to overload the * operator for matrix multiplication and how to overload the [] subscript operator for custom types.
You’ll also learn how to define prefix and postfix operators and how to overload the ?? operator for nil-coalescing. By the end of this section, you’ll have a deeper understanding of how to use operator overloading in Swift to improve the readability and expressiveness of your code.
Casting in Swift
The “Casting in Swift” section of the book covers the process of converting an instance of a type to another type. This is an important concept in Swift programming as it allows for maximum flexibility and ease of use, and is used in a variety of contexts. In this chapter, we will explore the different types of casting available in Swift, and how to implement them in your code to achieve the desired results.
This section will teach you type casting in Swift, including an overview of the concept, downcasting, type checking, and type casting for Any and AnyObject.
Type casting is an important aspect of Swift programming, allowing you to convert one type of object to another, check its type at runtime, and manage the type of objects in your code. Through understanding these techniques, you’ll be able to write more flexible and efficient code.
Coding & Decoding Data in Swift
The “Coding and Decoding Data in Swift” cookbook section is a comprehensive guide to working with data in Swift, specifically focusing on encoding and decoding data. This section covers topics such as Coding and Decoding Data in Swift, Decoding arrays and dictionaries in Swift and Handling Custom Keys and Missing Values while decoding in Swift. The first topic covers the basics of encoding and decoding data using the Codable protocol, including encoding and decoding basic data types such as strings, numbers, and booleans. The second topic discusses decoding arrays in Swift and the third topic focuses on handling custom keys and missing values while decoding.
This cookbook section is a must-read for developers who want to build robust and efficient data handling solutions in Swift.
Protocols in Swift Standard Library
Swift Standard Library offers a vast collection of protocols that provide a blueprint for defining the behavior of custom data types. These protocols offer a flexible and reusable approach to writing code and building custom types. In this section, you’ll be exploring the important protocols in the Swift Standard Library, including Equatable, Hashable, Comparable, and Codable, among others. We will dive into the syntax and implementation of each protocol and see how they can be utilized in real-world scenarios. Whether you are a beginner or an experienced developer, this section will provide you with a comprehensive guide on how to make the most out of the protocols in Swift Standard Library.