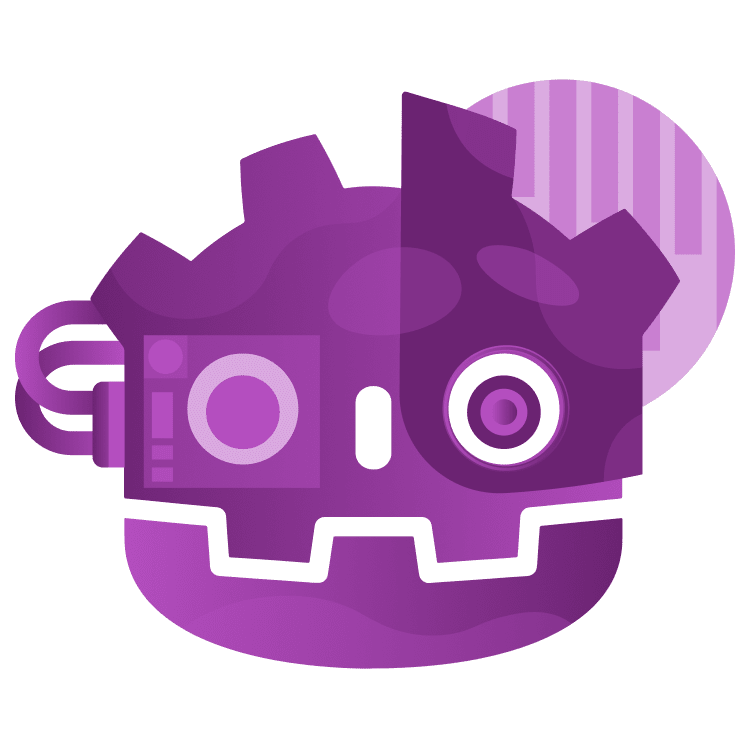
Extending the Editor with Plugins in Godot
Embark on a journey to harness the true power of Godot with editor plugins! Revolutionize your workflow with bespoke tools, efficient shortcuts, and personalized menu options. Delve deep into the art of plugin creation and unlock the boundless potential of Godot with ease. By Eric Van de Kerckhove.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Extending the Editor with Plugins in Godot
45 mins
- Getting Started
- Plugin Overview
- Creating Your First Plugin
- Scaffolding
- Taking a Closer Look
- Handling Node Selection
- Adding the Button
- Developing an Advanced Plugin
- Creating the Menu Scene
- Loading and Unloading the Menu
- Getting Selected Nodes
- 2D Physics in Godot
- Starting and Stopping Physics
- Custom Physics Spaces
- Physics Frames
- Adding Quality of Life Features
- Where to Go From Here?
Physics Frames
The Simulate 10 physics frames button will simulate 10 physics frames at a time and then stop. This is nice to slightly move your nodes without having to have perfect timing to hit the stop button.
You already have most of the logic in place, so adding the needed code is trivial. Replace the pass
keyword in the _on_ten_frames_pressed()
function with the following:
#1
_start_custom_physics()
# 2
for i in range(10):
await get_tree().physics_frame
# 3
_deactivate_custom_physics_space()
This function starts up the physics simulation, waits for 10 physics frames to pass and then stops the simulation. Here’s a rundown of the code:
- Start the physics simulation.
- This for-loop uses the
await
keyword to wait for thephysics_frame
signal ten times. This will stall the function for ten physics frames before moving on. - Deactivate the custom physics space and stop the physics simulation.
Now save the script, return to the 2D screen and click the Simulate 10 physics frames button while you have some nodes selected. You should see the nodes simulate for a short while before stopping.
Adding Quality of Life Features
While playing around with the plugin, you might have noticed that stopping the physics doesn’t mean that the velocities of the nodes get reset. If you make one of the balls roll to the side for example, and then put them on one of the platforms, it’ll keep its momentum and starts rolling. This is not always desirable.
To fix this potential issue, you need a quick way to reset the velocity of the selected nodes. To do this, replace the pass
keyword in the _on_reset_velocity_pressed()
function with:
# 1
var selected_nodes = _get_selected_nodes()
for selected_node in selected_nodes:
# 2
if selected_node is RigidBody2D:
# 3
selected_node = selected_node as RigidBody2D
# 4
selected_node.linear_velocity = Vector2.ZERO
selected_node.angular_velocity = 0
This gets all selected nodes and loops through them. If the node is a RigidBody2D
, it zeroes out the linear and angular velocity. Here’s the code in detail:
- Get all selected nodes and start iterating over them.
- If the selected node is a
RigidBody2D
… - Use the node as a
RigidBody2D
. This line isn’t crucial, but doing casts like these is a good idea as you’ll get better code completion from the code editor. - Reset the linear and angular velocity of the node to
Vector2.ZERO
and 0 respectively.
With this, you can reset the velocity of selected nodes with ease. Save the script and give it a shot! Make one of the ball move sideways by colliding with another node, then stop the simulation and reset the velocity. If you now the place the ball on a flat surface, it will stay put.
Where to Go From Here?
You can download the project files at the top or bottom of this tutorial by clicking the Download materials link.
Congratulations on making it to the end of the article. You’re now ready to start developing your own editor plugins and speed up your workflows. This is a useful skill to master as it can save you and your teammates a lot of time. I’ve only scratched the surface of plugins here, as you can make full-blown custom tools in Godot.
To find out more about creating plugins, check out Godot’s official documentation on the subject.
Now go out there and create your own plugins!
Thanks for reading. If you have any questions or feedback, please join the discussion below.