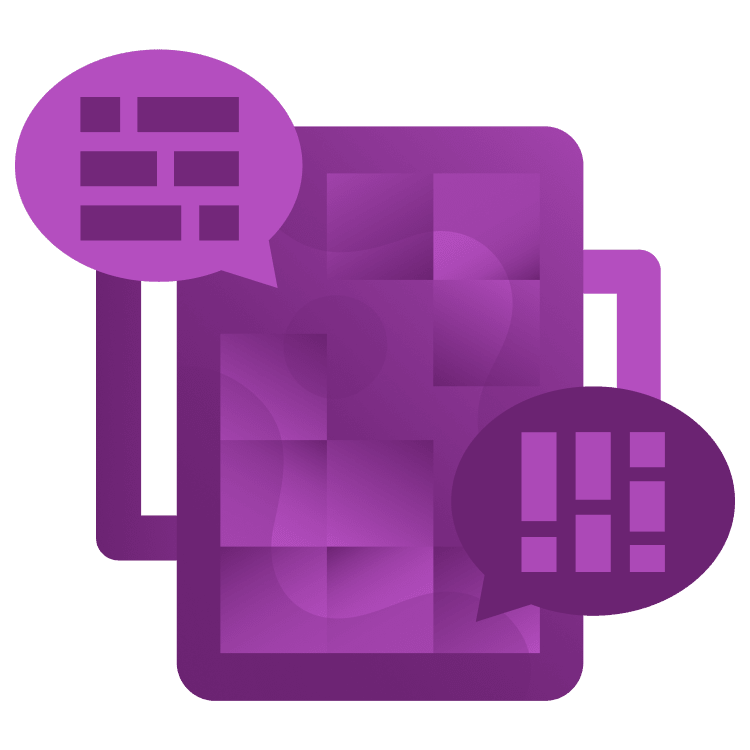
How to Translate Your Game Using the Unity Translation Package
Welcome to the wild world of localization, where Veggie Gladiators are about to prove that vegetables aren’t just for salads, but for speaking multiple languages too! Picture this: your game is a hit in Tokyo, and suddenly the Veggie Gladiators have to fight in a sushi bar. Without localization, they’ll be lost in translation faster than a potato in a fruit salad. So, let’s arm our Veggie Gladiators with languages and take over the global gaming arena, one veggie at a time! By Ben MacKinnon.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
How to Translate Your Game Using the Unity Translation Package
30 mins
- Getting Started
- Your First Translation
- Installing the Localization Package
- Adding Translation
- Building the Menu
- Adding a Translation Option Menu
- Setting Up Localization Manager
- Localization Settings
- Connecting Everything
- Finding Translations
- Importing Localization Files
- Translating Dialogue
- Translating the Interaction System
- Translating NPC Names
- Translating Questions
- Habla Español?
- Where to Go From Here?
Building the Menu
Before you add a new button to the menu, you should also add a translation for the New Game button. Can you repeat the previous steps for the Canvas ▸ Panel ▸ New Game Button ▸ Text (TMP) GameObject? The translation you need is Neues Spiel. If you get stuck, you can open the spoiler below for some help.
[spoiler title = "Solution"]
- Click the component menu for the TextMeshPro component and select Localize.
- Select the UI Strings table on the Table Collection dropdown on the new Localize String Event component.
- Click Add Table Entry.
- Update the values for the new entry:
- Entry Name: New Game
- English(en): New Game
- German(de): Neues Spiel
- Entry Name: New Game
- English(en): New Game
- German(de): Neues Spiel
Once you have the New Game button translated, duplicate the button by selecting it in the hierarchy and either right-click ▸ Duplicate or press Control-D. Name the new button Menu. Change the Y position of the button to 60 so that it sits just below the New Game button.
Select the Text (TMP) child of the Menu button. Update the string in the TextMeshPro component to read Menu.
Once more, update the Localization String Event by adding another Table Entry. Name this one Menu with the German translation being Menü.
Next, you’ll use this button to present a new panel with options for the user to change language.
Adding a Translation Option Menu
The translation options will be a new panel that’s presented in front of the title menu. When you’re done, it should look like this:
Select the Canvas gameObject in the hierarchy, and add a new GameObject ▸ UI ▸ Panel.
Name it OptionsPanel and set the color of the Image to 36, 36, 36, 221. This gives it a dark but slightly transparent background.
Select the Menu Button from earlier, and set its OnClick event to set the OptionsPanel GameObject.SetActive to true.
How the panel is laid out isn’t important, so feel free to make it look how you like. But for a speedy menu, select the Title gameObject and the two buttons from the original menu. Duplicate them, and drag the new objects to be children of the new OptionsPanel.
Rename the two buttons English and German. Update their text values to English and Deutsch and remove any LocalizeStringEvent components – you don’t want to translate these buttons!
Finally, to add translations to the new title, open up the Localization table, if you don’t still have it open, by selecting Window ▸ Asset Management ▸ Localization Tables. Click Add New Entry to create an entry with the Key value Choose Language. Set the English value to be the same and the German value to Sprache wählen.
Select the OptionsPanel ▸ Title object once more, and in the Localization String Event component, change the String Reference to this new key. You should also update the default value for the TextMeshPro component to Choose Language.
Now that you have some buttons for the different languages, it’s time to make them work!
Setting Up Localization Manager
In the RW ▸ Localization folder, create a new script called LocalizationManager. Once Unity recompiles, add an empty GameObject to the scene, also named LocalizationManager, and add the new script to the gameObject.
Now it’s time for a little scripting! Open the LocalizationManager script in your preferred code editor.
Localization Settings
Remember when I mentioned at the start that Unity saves the translation settings as a serialized asset? Well, that opens up being able to read and modify those settings from code! To change the language of the game, you only need one method, and the use of a LINQ query.
At the top of the LocalizationManager file, add the following using
statements:
using System.Linq;
using UnityEngine;
using UnityEngine.Localization.Settings;
Then, in the body of the class, add the following method:
public void SetLocale(string code)
{
// 1
var localeQuery = (from locale in LocalizationSettings.AvailableLocales.Locales
where locale.Identifier.Code == code
select locale).FirstOrDefault();
// 2
if (localeQuery == null)
{
Debug.LogError($"No locale for {code} found");
return;
}
// 3
LocalizationSettings.SelectedLocale = localeQuery;
}
Here’s what the code does:
- This line is a LINQ query. It’s searching all the
locales
you set up earlier and selecting the first one it finds that matches thecode
passed to the method. - This is a safety check to ensure you found a valid
locale
. - Finally, you update the
SelectedLocale
in theLocalizationSettings
to the one found by the query.
Save the script, and go back to the Unity editor.
Connecting Everything
Select the English button and replace the calls in On Click. First, you should drag LocalizationManager to the Object and select LocalizationManager ▸ SetLocale (string) as the call. Fill in the variable field with en – the code for the English Locale that you set at the beginning of the tutorial.
Second, add a reference to turn off the OptionsPanel gameObject. It should end up looking like this:
Finally, do the same with the German button, giving it the code de for the SetLocale method.
Turn the OptionsPanel gameObject off in the inspector, save the scene and click Play to try out the new menu options. You should be able to click Menu and then change the language between English and German.
You’ll notice that the dropdown you used to test the different language earlier also updates now when you use your UI to change the language.
Phew, that was a lot of work just to get a few buttons and title strings to update when changing language. But remember, there’s a whole host of dialogue strings to translate when the game actually starts!
Fortunately, there are some more tricks to use to mass import translation strings, and just a few edits to the existing code will have our Vegetables speaking German in no time!
Finding Translations
When working on a full production game supporting many languages, it isn’t feasible to manually step through every potential key and hand translate them all, unless you’re an extremely patient linguist! The usual approach for getting translations into your game would be to define all the keys you need and the source string. Then, you’d send your list of keys and sources off to a translator to provide all the translated strings back to reimport into your project.
Fortunately, the Unity Localization package supports a number of ways to export and import localized strings to your project. When dealing with an external or professional service, you’re likely to have your translation files hosted on the cloud with a service such as localizely or a self-hosted platform such as Weblate. These platforms can import and export files in formats such as CSV (Comma Separated Value) or XLIFF – an XML-based format that was created to be a standard for passing localizable data between applications.
In Unity, once you have created a Localization Table Collection like you did earlier, you can import or export that table of keys to either CSV or XLIFF from the component menu.
Of course, professional translation services don’t come free! If you’re just looking to get started and want a quick-win support solution, Unity Localization supports a third import method in the form of Google Sheets. The setup may be a little more complex, but the results can be instant.