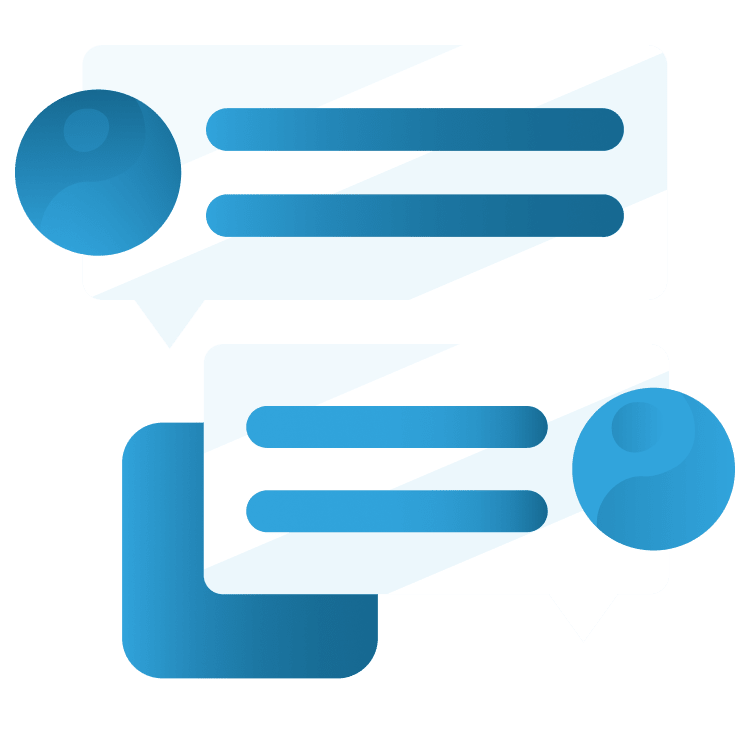
Live Chat With Pusher Using Provider
Learn how to setup a real-time messaging Flutter App using Pusher API and a Go backend deployed as a containerised web service to GCP Cloud Run. By Wilberforce Uwadiegwu.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Live Chat With Pusher Using Provider
30 mins
- Getting Started
- Setting up and Deploying the Back end
- Setting up Pusher
- Setting up Firebase
- Setting up GCP
- Deploying the Go Service
- Sending and Receiving Messages
- Configuring Pusher
- Receiving Messages
- Sending Messages
- Implementing UI
- Building the Messages Screen
- Adding An Input Field
- Adding the Messages View
- Building the Message Widget
- Supporting Images
- Displaying Images
- Where to Go From Here
Customer satisfaction could make or break a product. One way you could increase customer satisfaction is through a proper conflict resolution channel.
As a Software Engineer, you might not interact directly with customers, but you can build a channel for them to easily reach out to customer experience (CX) specialists and vice versa. In this tutorial, you’ll build Petplus, a mobile app for a veterinary company that doubles as an animal shelter. You’ll flesh out the real-time messaging functionality of the app, which will consist of two clients; one for users and the other for CX specialists. In this process, you’ll learn how to:
- Build complex interactive UIs.
- Build end-to-end messaging functionality.
- Deploy a containerized web service to GCP Cloud Run.
Getting Started
Download the project by clicking Download Materials at the top or bottom of this tutorial. Unzip the project, and you’ll find two folders: backend and mobile. Written in Go, the backend directory contains the code that’ll power the mobile app. Apart from deploying it, you won’t be interacting with it much.
The mobile directory is where you’ll work from; open it and open the starter folder inside with the latest version of Android Studio or Visual Studio Code. Part of the mobile app, like the API integration, is already complete so you can focus on the subject matter of this tutorial.
Open pubspec.yaml and click the Pub get tab that appears in your IDE. Open lib/main.dart and run the project to see this on your target emulator or device:
If you try to sign up, you’ll get an error because you still need to deploy the back end. You’ll do that in the next section.
Setting up and Deploying the Back end
In this section, you’ll set up Pusher, Firebase, and GCP. You’ll also deploy the files in the backend directory to GCP Cloud Run. Pusher provides a hosted pub/sub messaging API called Channels. This API lets the Petplus app create and listen to events on a channel and then act upon them directly. The app will implement each customer service message as an event, thus creating a real-time messaging functionality between clients. GCP describes Cloud Run as a “serverless compute platform that abstracts away all infrastructure management, so you can focus on what matters most — building great applications.”
Setting up Pusher
Pusher will power the real-time messaging back end for the apps. Go to Pusher and sign up. After signup, click “Get Started” on the setup page:
Next, complete the Channels set up by filling in the form like so:
Finally, scroll down to Step 2 on the page and note down the following values: AppID, Key, Secret and Cluster:
Setting up Firebase
You’ll use Firebase for user account management and persisting user messages.
Follow steps 1 and 2 on this page to set up Firebase Project and enable Firebase Authentication. Note that Google authentication is not required.
Next, click Firestore Database from the left pane on the Firebase Console under the Build section. Enable the database.
Lastly, click the Indexes tab and create a composite index like shown below:
When fetching the message history, the web service orders the query by the sentAt field; hence you created an index so Firestore can process the query.
Setting up GCP
Once you’ve finished with Firebase, you have to set up GCP for the same project. The web service uses two core GCP services: Cloud Run and Cloud Storage. You’ll deploy the web service to Cloud Run, and the images uploaded by users in messages will be hosted on Cloud Storage. What’ll this cost you? If you follow the steps in this tutorial exactly, you should stay within the free tier, so it’s free. Well, free to you; Google is picking up the bill!
Now, open GCP Console. Accept the terms and conditions if you still need to do so. Select the Firebase project you created earlier and enable billing for it. For new accounts, you might be eligible for a free trial; enable it.
Deploying the Go Service
Now, you’ll build and deploy the web service app. The complexities of the deployment process have been abstracted into a bespoke Makefile to enable easier facilitation. So you only have to run two make
commands to deploy. However, you have to install some software:
- Golang: the web service is written in Go; hence it’s needed to compile it.
- Docker: to containerize the Go app before deploying it with gcloud. Start Docker after the installation.
- gcloud cli: to deploy the Docker container to cloud Run.
- yq: to parse the YAML configuration in the Makefile.
Next, fill in the config file. Inside the folder you unzipped earlier, using any text editor, open the config.yaml file inside backend directory. Fill it like so:
-
port
: Leave this empty; it’ll be read from Cloud Run’s environment variables. -
gcpProject
: The Firebase or GCP project id. You can find it in the Firebase project settings. -
messageImagesBucket
: The name of the bucket where images from messages will be stored. You can choose a name yourself using these guidelines. -
pusherId
: Pusher AppId from previous step. -
pusherKey
: Pusher key from previous step. -
pusherSecret
: Pusher Secret from previous step. -
pusherCluster
: Pusher Cluster from previous step. -
firebaseAPIKey
: Firebase Web API key. You can find it in the Firebase project settings, like the Firebase project id.
Inside the backend directory is a Makefile; this is the deploy script. Using Terminal, run these commands sequentially from this directory:
-
make setup-gcp
: creates the storage bucket with the name you filled in above and enables Cloud Run for the project. -
make deploy
: builds and deploys the docker container to Cloud Run.
If both commands complete successfully, you’ll see this on the command line:
The mobile app needs the service URL, so copy it.
Good job on completing this step!
Sending and Receiving Messages
In the previous section, you deployed the Go service and got the service URL. In this section, you’ll set up Pusher on the mobile and implement the messaging functionality.